Django best practices: Project structure, code-writting, static files tips and more
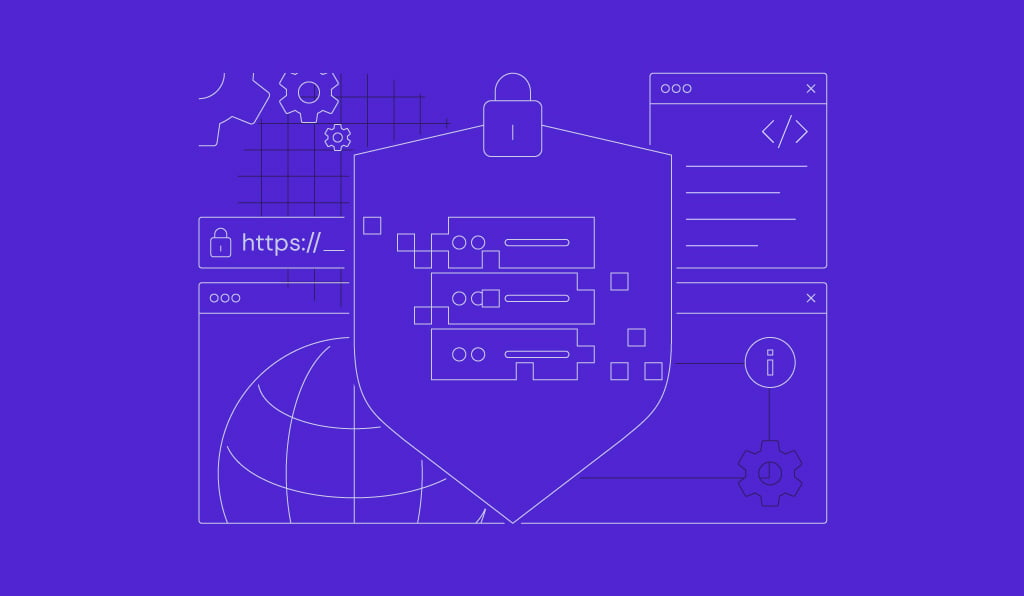
Django is the ideal solution for building complex web applications since it is simple and easy to use. However, you have to apply the best development practices while creating Django projects to keep the code clean and the structure effective and extensible.
This guide covers key practices for Django application development, including efficient import management, clear internal coordination, and best practices for secure, high-performance applications on Linux VPS.
These techniques will simplify development and help you build scalable and easy-to-maintain Django applications.
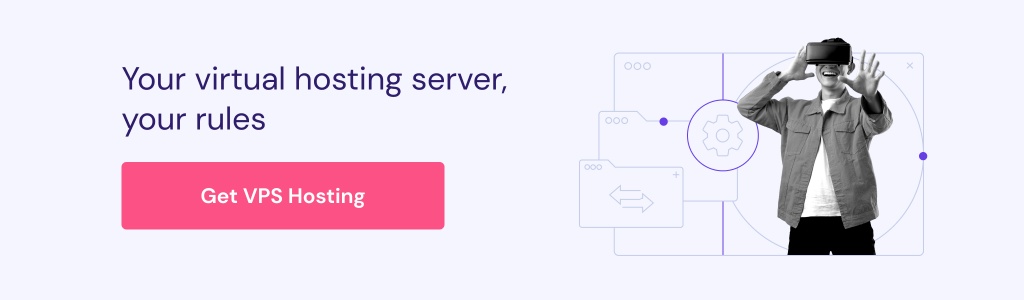
Django project structure and organization tips
Use the default Django project layout
Django comes with a default project structure which is helpful in most scenarios. This structure separates core configurations, like settings, URL routing, and deployment files, into distinct files, promoting organization and maintainability.
However, the default layout does not include directories for templates, static files, or apps, which developers must create based on the project’s needs. This layout works well for small projects, but as the complexity increases, the structure may need adjustments.

In larger projects, it’s often beneficial to divide settings into multiple files, such as base, development, and production settings, instead of placing everything in a single settings.py file. This approach makes setting management easier and provides more control over configurations.
Additionally, grouping related apps or functionalities helps create a more organized code structure and improves modularity. Organizing static files, including templates, by individual apps rather than relying on a single, large app will also make the codebase easier to maintain and navigate.

As your Django project grows, make sure to back up your work regularly using version control systems like Git to avoid unintentional code loss or disorganization. Avoid overly complex, nested structures and excessive folders below the root level. Instead, create dedicated folders for logs, media files, and custom scripts to keep things organized.
By planning your Django project structure from the start, you can create a scalable and easy-to-manage codebase that adapts to the project’s evolving demands. This organized approach simplifies testing, deployment, and future updates, making the project easier to maintain in the long term.
For more information on structuring your project, refer to Django Project Structure.
Create an apps directory for better organization
In a typical Django development workflow, developers often set up an apps directory within the project to house all Django applications. This approach helps keep the root directory clean and organized, especially as the project grows with additional apps, static files, and other components.
Static files and templates for each app should be stored within their respective app directories or in a centralized location, depending on the project’s needs.
Organizing apps in a dedicated folder not only improves the overall structure of the project but also reduces clutter, making it easier to locate files and maintain the codebase.
When creating apps, it is vital to ensure that no app is capable of doing more than its primary function. This means that each app should only serve a single purpose and do it well.
For example, you may need to create a separate app for authorization, another for blogs, and another for payments. If you manage your app directory well, you may be able to take unused apps from other projects and use them for your current project with minor modifications.

Also, a setup like this optimizes how people work together. When you are part of a team, for instance, every developer can concentrate on their particular app without stressing about how that will interfere with other aspects of the project.
In addition, it makes testing and deployment easier as the particular application can be treated as an independent unit.
All in all, the apps directory benefits the management of the code base of a Django project and improves organization, reuse, and expansion.
Separate settings files for different environments
Managing different settings for development, staging, and production environments is crucial to ensure your Django project runs smoothly and securely in each phase. One widely recommended practice is to split your settings into multiple files, such as base.py, development.py, and production.py. To learn more, check out Splitsetting.

Alt Text: Example of split settings files in Django for managing different environments securely
When sensitive data is not stored within version control, it is less likely that such information will be leaked unintentionally. This way, you may use environment variables featured in the production.py setting file to save vital information, which is easily updated without modifying the code itself.
Even certain tools, such as django-environ, also offer support for environment variable management. It is a best practice to keep sensitive data, such as secret keys, database passwords, or API tokens, in environment variables.
Additional tools, such as python-decouple, allow for the easy storage and retrieval of these variables, thereby relieving the difficulty of embedding sensitive details in source code.
For a deeper dive into managing settings, check out Managing Django Settings.
Django code-writing best practices
Writing clean code is essential for easy maintenance. Follow Django’s coding standards and Python’s PEP8 guidelines to ensure that your code is easy to read and maintain.
Tools like flake8 and black can automatically check and format your code, ensuring that it adheres to these standards. While Function-Based Views (FBVs) are easier for beginners to grasp, Class-Based Views (CBVs) organize code better, making it more reusable and extendable.
With CBVs, you can also take advantage of Django’s object-oriented features and make your Django views more modular. For example, CBVs like ListView, DetailView, and CreateView save you from writing repetitive code for common operations.
Also, write meaningful comments, use clear naming conventions, and avoid deeply nested logic to maintain a clean and efficient codebase.
Example of a Class-Based View:
from django.views.generic import ListView from .models import Post class PostListView(ListView): model = Post template_name = 'blog/post_list.html' context_object_name = 'posts'
Modularize code with Django apps
The application architecture of Django promotes modularity. Every app should contain one specific scope of functionality of your project, making it less complicated and more expandable.
For instance, in an eCommerce application, you could have separate apps for products, orders, and customers. This would help maintain your project easier and allow you to use the apps in other projects.
Django signals are useful because they allow different parts of your application to be notified when specific events occur. However, overusing signals can lead to confusion. It’s best to reserve them for important events, such as sending a confirmation email when a user signs up.
Django models and database best practices
Plan your models so that they are efficient and can scale as needed. Define adequate field types for your data and use indexes to make your queries effective.
For example, adding an index can improve query speed if a field is searched or filtered frequently. However, be mindful that excessive indexing can impact write operations, so use indexes sparingly.
You can create custom managers and query sets to simplify complex queries, making them reusable throughout the project. This way, your code becomes more descriptive, and there is also little to no code duplication.
Example of a custom manager:
class PublishedManager(models.Manager): def get_queryset(self): return super().get_queryset().filter(status='published')
Avoid N+1 query problems
The N+1 query problem occurs when a database query is executed once for each object in a queryset, leading to performance issues. To avoid this, use Django’s select_related and prefetch_related methods to fetch related objects in a single query:
- select_related – optimizes single-valued relationships like ForeignKey and OneToOneField.
- prefetch_related – ideal for multi-valued relationships like ManyToManyField or reverse ForeignKey.
Example:
posts = Post.objects.select_related('author').all()
Use migrations properly
Migrations are vital for maintaining your database schema. Make sure your migrations are:
- Minimal – avoid generating unnecessary migrations by reviewing changes carefully before creating them.
- Well-reported – see descriptive migration names and document complex changes to make them easier to understand and manage.
- Tested – always test migrations locally and in a staging environment before applying them in production.
Following these practices will prevent potential problems during production rollouts.
Django security best practices
Always keep Django and third-party dependencies up to date to avoid security vulnerabilities. Tools like pip-tools can help you manage your dependencies and ensure that everything stays up to date.
Django comes with several built-in security features, such as XSS and CSRF protection, clickjacking prevention, and secure sessions. Ensure that these are enabled and configured properly in your project to protect against common web vulnerabilities.

Use Django’s built-in authentication system to enforce strong password policies. You can also customize password validation to ensure that users choose secure passwords.
Ensure that sensitive data, such as user passwords and API tokens, are encrypted. Use libraries like django-cryptography for encrypting sensitive fields and configure your production environment to use HTTPS. Learn more in the official guide on Django Security Best Practices.
Django template usage tips
Django templates should contain mainly presentations and not business rules. Avoid repeating details in your templates for better accuracy by using Django’s built-in template tags and filters for more advanced tasks.

Django lets you structure templates so that common elements, like headers and footers, are stored in one file, which other templates can extend. This reduces duplication and makes managing your templates easier.
You can also improve your application’s performance by using caching. Django offers per-site, per-view, and low-level caching to reduce template load times and minimize server strain.
Django static and media file management tips
Properly organizing your static and media files helps streamline the development process. Keep them in designated directories (static/ for static files and media/ for user-uploaded files) to avoid clutter. What’s more, serving static files through a Content Delivery Network (CDN) can significantly improve load times and user experience.

Consider using a CDN, like AWS CloudFront or Cloudflare, to manage your static files in production. Optimizing images and static files reduces bandwidth usage and improves performance. Tools like django-compressor and whitenoise can help you compress and serve static files more efficiently.
Django testing and debugging best practices

Alt Text: Write Tests for Your Code
Testing is essential for ensuring that your code works as expected. Write unit tests for models, views, forms, and templates using Django’s testing framework. This will help you catch bugs early and ensure that your app remains reliable.
In addition to Django’s built-in testing framework, tools like pytest and coverage.py can enhance your testing process by providing more advanced features and better reporting.
Django provides several debugging tools, such as django-debug-toolbar, which can help you identify performance bottlenecks and debug SQL queries. Use these tools to optimize your application’s performance, but remember to disable them in production for security and efficiency.
Django performance optimization tips

Caching is one of the most effective ways to improve performance. Use Django’s built-in caching framework to cache database queries, views, and templates. Popular caching backends include Memcached and Redis.

Middleware can add overhead to your requests. Review your middleware stack and remove any unused or non-essential middleware to reduce request processing time.
Reducing the number of database hits is crucial for performance. Use techniques like caching, batch processing, and efficient query patterns to minimize database interactions.
Django deployment best practices
When deploying your Django app, use tools like Docker, Heroku, or AWS Elastic Beanstalk for scalable and reliable deployments. These tools make it easier to manage your application in production.

Automate deployment with CI/CD
Automating your deployment process using Continuous Integration/Continuous Deployment (CI/CD) tools like GitHub Actions or Jenkins ensures that your app is tested and deployed efficiently.
These tools can automate tasks like running tests, building the application, and deploying it to production, reducing manual intervention and improving consistency.
Monitor application performance and errors
Application performance monitoring helps you to diagnose and fix problems more promptly. Use tools like Sentry for error tracking and New Relic for comprehensive performance monitoring, including transaction traces and database insights.
Configure alerts to receive real-time notifications of critical issues, and combine these tools with Django’s built-in logging for a complete monitoring strategy.
Conclusion
Following the best practices of the Django framework enables developers to build scalable and efficient web applications.
Organizing related files into separate folders in Django’s default layout enhances the clarity and simplicity of the codebase, starting from the project’s structure. base.py, development.py, and production.py files contribute to the project’s security and flexibility, with sensitive data securely managed using environment variables and tools like django-environ.
Database and performance optimization are equally important. Planning models with proper field types and indexing speeds up database queries while avoiding common pitfalls like the N+1 query problem through methods like select_related and prefetch_related.
Performance optimization practices like caching strategies on various levels (site-wide, per-view, or template-level) and sending static files via CDN enhance user experience and lower the server load. Also, tools such as Django-compressor and whitenoise enhance this process.
In summary, the practices explained in this article ensure that Django applications meet current requirements and overcome future challenges. Developers following these practices will be able to easily deliver high-quality projects that excel in functionality, performance, and maintainability.